Configuración para empezar
Central de mensajes es una solución de CPaaS con un conjunto de productos que incluye API de SMS OTP, API de SMS y la plataforma de marketing de WhatsApp.
Deberás crear una cuenta en Message Central, p. ej. regístrate gratis. Recibirás un identificador de cliente que utilizarás en tu solicitud.
Instalación de Java 8+
- Descargar Java:
Visite el sitio web oficial de Java (https://www.java.com/en/download/) y descargue la versión 8 o posterior del Kit de desarrollo de Java (JDK) para su sistema operativo.
- Ejecute el instalador:
Una vez finalizada la descarga, ejecuta el archivo de instalación. Siga las instrucciones que aparecen en pantalla para instalar Java en su sistema.
- Verifique la instalación:
Abra la línea de comandos (en Windows) o la terminal (en macOS/Linux) y ejecute el siguiente comando para verificar la instalación:
java -version
Esto debería imprimir la versión de Java instalada. Si Java está instalado correctamente, debería ver algo similar a:
java version "1.8.0_261"
Java(TM) SE Runtime Environment (build 1.8.0_261-b12)
Java HotSpot(TM) 64-Bit Server VM (build 25.261-b12, mixed mode)
Si obtiene una versión diferente (por ejemplo, Java 11 o posterior), está bien siempre que la versión sea 8 o superior.
- Configure JAVA_HOME (opcional):
Es posible que algunas aplicaciones y herramientas requieran que defina la variable de entorno JAVA_HOME. Para configurarla, sigue las instrucciones de tu sistema operativo:
Ventanas:
Haga clic con el botón derecho en «Mi PC» y seleccione «Propiedades» > «Configuración avanzada del sistema» > «Variables de entorno».
En «Variables del sistema», haga clic en «Nuevo» y cree una nueva variable denominada JAVA_HOME con el valor establecido en el directorio de instalación de Java (por ejemplo, C:\Program Files\ Java\ jdk1.8.0_261).
MacOS/Linux:
Abre un editor de texto y crea o edita el archivo.bash_profile en tu directorio principal. Añada la siguiente línea y sustituya /path/to/java por la ruta real a la instalación de Java:
export JAVA_HOME=/path/to/java
Guarda el archivo y reinicia el terminal para que los cambios surtan efecto.
Tras seguir estos pasos, debe tener Java 8 o una versión posterior instalada y lista para usarse en su sistema.
Pasos de integración
Este proceso implica los siguientes tres pasos importantes:
- Detalles de Message Central
- Agregar detalles en el código
- Enviar un SMS OTP de prueba
a. Detalles de la central de mensajes
Tras crear una cuenta en Message Central, necesitará los siguientes detalles:
- ID de cliente: puedes obtener el ID de cliente en la consola principal de Message Central
- Credenciales de inicio de sesión: necesitarás un correo electrónico y tendrás que crear una contraseña.
b. Agregar detalles en el código
Para agregar detalles de MessageCentral en el código, busque la definición de la variable con el nombre «customerID», «correo electrónico» y «contraseña»:
private static final String customerID = "C-45539F39A44****";
private static final String email = "abhishek****k68@gmail.com";
private static final String password = "*****";
private static final String baseURL = "https://cpaas.messagecentral.com";
private static String authToken;
private static String verificationId;
Ahora, necesitas crear un archivo JAVA es decir, Main.java: Y añada el código proporcionado a este archivo.
package u2opia.message central.verification;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.InetSocketAddress;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpServer;
import org.json.JSONObject;
public class Main {
private static final int PORT = 3000;
private static final String customerID = "C-45539F39A449437";
private static final String email = "abhishekkaushik68@gmail.com";
private static final String password = "Wesee@123";
private static final String baseURL = "https://cpaas.messagecentral.com";
private static String authToken;
private static String verificationId;
private static String parseJSON(String jsonString, String key) {
try {
JSONObject jsonObject = new JSONObject(jsonString);
if (jsonObject.has(key)) {return jsonObject.getString(key);}
} catch (Exception e) {e.printStackTrace();}
return null;
}
private static String generateAuthToken() {
try {
String base64String = Base64.getEncoder().encodeToString(password.getBytes(StandardCharsets.UTF_8));
String url = baseURL + "/auth/v1/authentication/token?country=IN&customerId=" + customerID + "&email=" + email + "&key=" + base64String + "&scope=NEW";
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("GET");
con.setRequestProperty("Accept", "*/*");
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
authToken = parseJSON(response, "token");
return authToken;
} else {System.out.println("Error generating auth token. Response code: " + responseCode);}
} catch (IOException e) {e.printStackTrace();}
return null;
}
private static String sendOtp(String countryCode, String mobileNumber) {
try {
String url = baseURL + "/verification/v3/send?countryCode=" + countryCode + "&customerId=" + customerID + "&flowType=SMS&mobileNumber=" + mobileNumber;
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Accept", "*/*");
con.setRequestProperty("authToken", authToken);
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
verificationId = parseJSON(parseJSON(response,"data"), "verificationId");
return verificationId;
} else {System.out.println("Error sending OTP. Response code: " + responseCode);}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
private static String validateOtp(String otpCode, String countryCode, String mobileNumber) {
try {
String url = baseURL + "/verification/v3/validateOtp?countryCode=" + countryCode + "&mobileNumber=" + mobileNumber + "&verificationId=" + verificationId + "&customerId=" + customerID + "&code=" + otpCode;
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("GET");
con.setRequestProperty("Accept", "*/*");
con.setRequestProperty("authToken", authToken);
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
String verificationStatus = parseJSON(response, "verificationStatus");
return verificationStatus;
} else {System.out.println("Error validating OTP. Response code: " + responseCode);}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
public static void main(String[] args) {
HttpServer server;
try {server = HttpServer.create(new InetSocketAddress(PORT), 0);}
catch (IOException e) {throw new RuntimeException(e);}
server.createContext("/sendotp/", new SendOtpHandler());
server.createContext("/validateOtp/", new ValidateOtpHandler());
server.start();
System.out.println("Server running at http://localhost:" + PORT);
}
static class SendOtpHandler implements HttpHandler {
@Override
public void handle(HttpExchange exchange) throws IOException {
if (exchange.getRequestMethod().equalsIgnoreCase("POST")) {
String[] params = exchange.getRequestURI().getPath().split("/");
String countryCode = params[2];
String mobileNumber = params[3];
generateAuthToken();
verificationId = sendOtp(countryCode, mobileNumber);
if (verificationId != null) {
String responseBody = "Otp sent! Successfully";
exchange.sendResponseHeaders(200, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
} else {
String responseBody = "Bad Request";
exchange.sendResponseHeaders(400, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
} else {
String responseBody = "Method not allowed";
exchange.sendResponseHeaders(405, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
}
}
static class ValidateOtpHandler implements HttpHandler {
@Override
public void handle(HttpExchange exchange) throws IOException {
if (exchange.getRequestMethod().equalsIgnoreCase("GET")) {
String[] params = exchange.getRequestURI().getPath().split("/");
String countryCode = params[2];
String mobileNumber = params[3];
String otpCode = params[4];
generateAuthToken();
String verificationStatus = validateOtp(otpCode, countryCode, mobileNumber);
if (verificationStatus != null && verificationStatus.equals("VERIFICATION_COMPLETED")) {
String responseBody = "Otp verification Done!";
exchange.sendResponseHeaders(200, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
} else {
String responseBody = "Bad Request";
exchange.sendResponseHeaders(400, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
} else {
String responseBody = "Method not allowed";
exchange.sendResponseHeaders(405, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
}
}
}
c. Enviar un SMS OTP de prueba
Si necesita probar el servicio sin código, puede ir a verificación por SMS gratuita página en el sitio web de Message Central.
Para garantizar que la integración se realice correctamente, envíe un SMS OTP de prueba de la siguiente manera:
1. Ejecute el código JAVA mediante el comando
Compila tu archivo Java con el comando javac.
javac Main.java
Ejecute su programa Java con el comando java
java Main
2. Abra el Postman y configure el método de solicitud como POST y
URL como http://localhost:3000/sendotp/<countryCode>/<phone_number>
Aquí está el puerto 3000, es predeterminado y está definido en código, se puede cambiar.
Por ejemplo, para la URL de un número de teléfono indio: http://localhost:3000/sendotp/91/123****123
- Imagen 1: Sending Otp
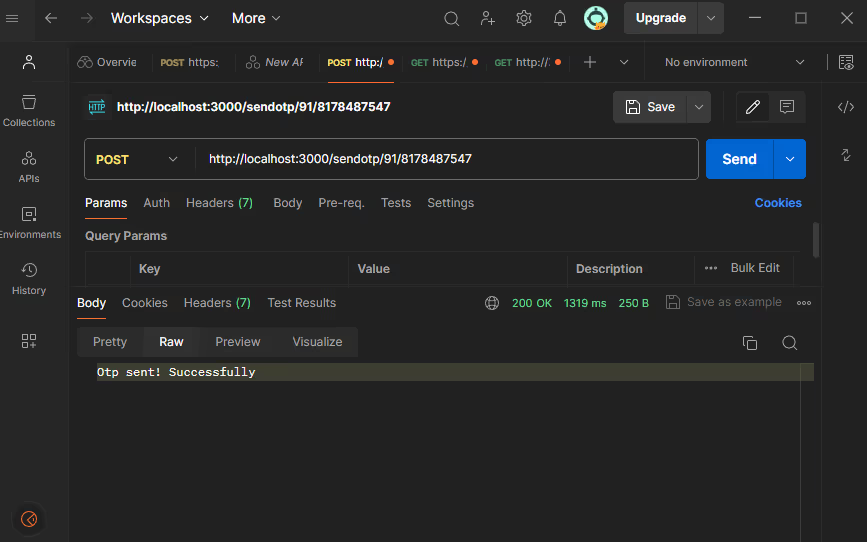
3. Ahora tienes una OTP en tu bandeja de entrada de SMS. Pruebe su propia API de validación de OTP para validar OTP.
Abra Postman y configure el método de solicitud como GET y
URL como http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Aquí está el puerto 3000, es predeterminado y está definido en código, se puede cambiar.
Por ejemplo, para la URL del número de teléfono indio: http://localhost:3000/validateOtp/91/123****123/ ****
- Imagen 2: Verificación de Otp
