Setup to Get Started
Message Central is a CPaaS solution with a suite of products including OTP SMS APIs, SMS APIs and WhatsApp marketing platform.
You’d need to create an account with Message Central i.e. sign up for free. You will receive a customer ID, which you will use in your application.
Installing Java 8+
- Download Java:
Visit the official Java website (https://www.java.com/en/download/) and download the Java Development Kit (JDK) version 8 or later for your operating system.
- Run the Installer:
Once the download is complete, run the installer file. Follow the on-screen instructions to install Java on your system.
- Verify Installation:
Open the command prompt (on Windows) or terminal (on macOS/Linux) and run the following command to verify the installation:
java -version
This should print the installed Java version. If Java is installed correctly, you should see something similar to:
java version "1.8.0_261"
Java(TM) SE Runtime Environment (build 1.8.0_261-b12)
Java HotSpot(TM) 64-Bit Server VM (build 25.261-b12, mixed mode)
If you get a different version (e.g., Java 11 or later), that's fine as long as the version is 8 or higher.
- Set JAVA_HOME (Optional):
Some applications and tools may require you to set the JAVA_HOME environment variable. To set it, follow the instructions for your operating system:
Windows:
Right-click on "My Computer" and select "Properties" > "Advanced System Settings" > "Environment Variables".
Under "System Variables", click "New" and create a new variable named JAVA_HOME with the value set to the installation directory of Java (e.g., C:\Program Files\Java\jdk1.8.0_261).
macOS/Linux:
Open a text editor and create or edit the .bash_profile file in your home directory. Add the following line, replacing /path/to/java with the actual path to your Java installation:
export JAVA_HOME=/path/to/java
Save the file and restart the terminal for the changes to take effect.
After following these steps, you should have Java 8 or a later version installed and ready to use on your system.
Integration Steps
This process involves the following three significant steps:
- Message Central details
- Adding details in code
- Send a test OTP SMS
a. Message Central Details
After creating an account on Message Central, you need the following details:
- Customer Id - You can get the customer ID from Message Central home console
- Login Credentials: You’d require an email and would need to create a password.
b. Adding Details in Code
To add MessageCentral details on the code find out the variable definition with name “customerId”, “email” and “password”:
private static final String customerID = "C-45539F39A44****";
private static final String email = "abhishek****k68@gmail.com";
private static final String password = "*****";
private static final String baseURL = "https://cpaas.messagecentral.com";
private static String authToken;
private static String verificationId;
Now, you need to create a JAVA file i.e Main.java: And add provided code into this file.
package u2opia.message central.verification;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.InetSocketAddress;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpServer;
import org.json.JSONObject;
public class Main {
private static final int PORT = 3000;
private static final String customerID = "C-45539F39A449437";
private static final String email = "abhishekkaushik68@gmail.com";
private static final String password = "Wesee@123";
private static final String baseURL = "https://cpaas.messagecentral.com";
private static String authToken;
private static String verificationId;
private static String parseJSON(String jsonString, String key) {
try {
JSONObject jsonObject = new JSONObject(jsonString);
if (jsonObject.has(key)) {return jsonObject.getString(key);}
} catch (Exception e) {e.printStackTrace();}
return null;
}
private static String generateAuthToken() {
try {
String base64String = Base64.getEncoder().encodeToString(password.getBytes(StandardCharsets.UTF_8));
String url = baseURL + "/auth/v1/authentication/token?country=IN&customerId=" + customerID + "&email=" + email + "&key=" + base64String + "&scope=NEW";
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("GET");
con.setRequestProperty("Accept", "*/*");
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
authToken = parseJSON(response, "token");
return authToken;
} else {System.out.println("Error generating auth token. Response code: " + responseCode);}
} catch (IOException e) {e.printStackTrace();}
return null;
}
private static String sendOtp(String countryCode, String mobileNumber) {
try {
String url = baseURL + "/verification/v3/send?countryCode=" + countryCode + "&customerId=" + customerID + "&flowType=SMS&mobileNumber=" + mobileNumber;
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Accept", "*/*");
con.setRequestProperty("authToken", authToken);
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
verificationId = parseJSON(parseJSON(response,"data"), "verificationId");
return verificationId;
} else {System.out.println("Error sending OTP. Response code: " + responseCode);}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
private static String validateOtp(String otpCode, String countryCode, String mobileNumber) {
try {
String url = baseURL + "/verification/v3/validateOtp?countryCode=" + countryCode + "&mobileNumber=" + mobileNumber + "&verificationId=" + verificationId + "&customerId=" + customerID + "&code=" + otpCode;
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("GET");
con.setRequestProperty("Accept", "*/*");
con.setRequestProperty("authToken", authToken);
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
String verificationStatus = parseJSON(response, "verificationStatus");
return verificationStatus;
} else {System.out.println("Error validating OTP. Response code: " + responseCode);}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
public static void main(String[] args) {
HttpServer server;
try {server = HttpServer.create(new InetSocketAddress(PORT), 0);}
catch (IOException e) {throw new RuntimeException(e);}
server.createContext("/sendotp/", new SendOtpHandler());
server.createContext("/validateOtp/", new ValidateOtpHandler());
server.start();
System.out.println("Server running at http://localhost:" + PORT);
}
static class SendOtpHandler implements HttpHandler {
@Override
public void handle(HttpExchange exchange) throws IOException {
if (exchange.getRequestMethod().equalsIgnoreCase("POST")) {
String[] params = exchange.getRequestURI().getPath().split("/");
String countryCode = params[2];
String mobileNumber = params[3];
generateAuthToken();
verificationId = sendOtp(countryCode, mobileNumber);
if (verificationId != null) {
String responseBody = "Otp sent! Successfully";
exchange.sendResponseHeaders(200, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
} else {
String responseBody = "Bad Request";
exchange.sendResponseHeaders(400, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
} else {
String responseBody = "Method not allowed";
exchange.sendResponseHeaders(405, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
}
}
static class ValidateOtpHandler implements HttpHandler {
@Override
public void handle(HttpExchange exchange) throws IOException {
if (exchange.getRequestMethod().equalsIgnoreCase("GET")) {
String[] params = exchange.getRequestURI().getPath().split("/");
String countryCode = params[2];
String mobileNumber = params[3];
String otpCode = params[4];
generateAuthToken();
String verificationStatus = validateOtp(otpCode, countryCode, mobileNumber);
if (verificationStatus != null && verificationStatus.equals("VERIFICATION_COMPLETED")) {
String responseBody = "Otp verification Done!";
exchange.sendResponseHeaders(200, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
} else {
String responseBody = "Bad Request";
exchange.sendResponseHeaders(400, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
} else {
String responseBody = "Method not allowed";
exchange.sendResponseHeaders(405, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
}
}
}
c. Send a Test OTP SMS
If you need to test the service without code, you can go to the free SMS verification page on the Message Central’s website.
To ensure that the integration is successful, send a test OTP SMS as follows:
1. Run the JAVA code using command
Compile your Java file using the javac command.
javac Main.java
Run your Java program using the java command
java Main
2. Open the Postman and set Request Method as POST and
URL as http://localhost:3000/sendotp/<countryCode>/<phone_number>
Here’s the port 3000, it is default and defined in code, can be changed.
Example, For Indian Phone Number URL : http://localhost:3000/sendotp/91/123****123
- Image 1 : Sending Otp
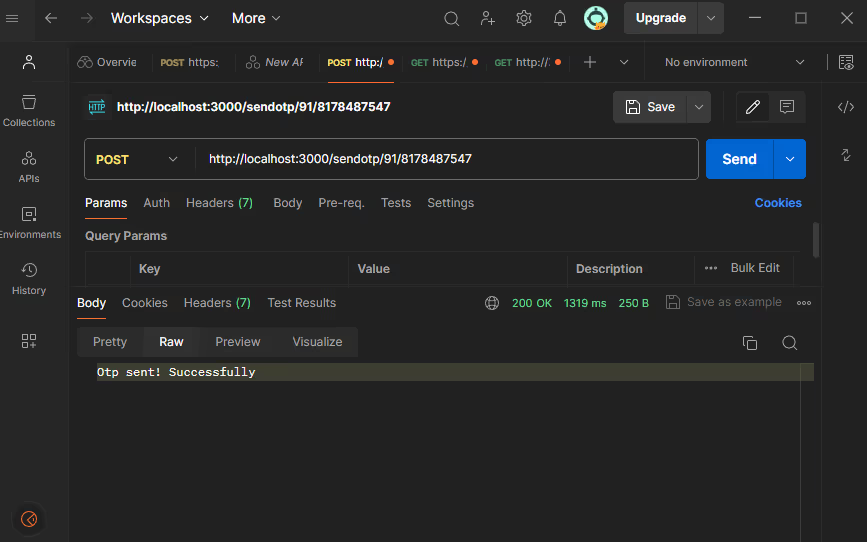
3. Now You have an otp on your sms inbox. Try your own validation Otp API to validate OTP.
Open the Postman and set Request Method as GET and
URL as http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Here’s the port 3000, it is default and defined in code, can be changed.
Example, For Indian Phone NumberURL : http://localhost:3000/validateOtp/91/123****123/****
- Image 2 : Verifying Otp
