Setup untuk Memulai
Pusat Pesan adalah solusi CPaaS dengan rangkaian produk termasuk API SMS OTP, API SMS dan platform pemasaran WhatsApp.
Anda harus membuat akun dengan Message Central yaitu Mendaftar gratis. Anda akan menerima ID pelanggan, yang akan Anda gunakan dalam aplikasi Anda.
Menginstal Java 8+
- Unduh Java:
Kunjungi situs web resmi Java (https://www.java.com/en/download/) dan unduh Java Development Kit (JDK) versi 8 atau yang lebih baru untuk sistem operasi Anda.
- Jalankan Installer:
Setelah unduhan selesai, jalankan file penginstal. Ikuti petunjuk di layar untuk menginstal Java pada sistem Anda.
- Verifikasi Instalasi:
Buka command prompt (pada Windows) atau terminal (di MacOS/Linux) dan jalankan perintah berikut untuk memverifikasi instalasi:
java -version
Ini harus mencetak versi Java yang diinstal. Jika Java diinstal dengan benar, Anda akan melihat sesuatu yang mirip dengan:
java version "1.8.0_261"
Java(TM) SE Runtime Environment (build 1.8.0_261-b12)
Java HotSpot(TM) 64-Bit Server VM (build 25.261-b12, mixed mode)
Jika Anda mendapatkan versi yang berbeda (misalnya, Java 11 atau lebih baru), tidak apa-apa selama versinya 8 atau lebih tinggi.
- Atur JAVA_HOME (Opsional):
Beberapa aplikasi dan alat mungkin mengharuskan Anda untuk mengatur variabel lingkungan JAVA_HOME. Untuk mengaturnya, ikuti instruksi untuk sistem operasi Anda:
Jendela:
Klik kanan pada “My Computer” dan pilih “Properti"> “Advanced System Settings” > “Environment Variables”.
Di bawah “Variabel Sistem”, klik “Baru” dan buat variabel baru bernama JAVA_HOME dengan nilai yang ditetapkan ke direktori instalasi Java (misalnya, C:\Program Files\ Java\ jdk1.8.0_261).
MacOS/Linux:
Buka editor teks dan buat atau edit file.bash_profile di direktori home Anda. Tambahkan baris berikut, ganti /path/to/java dengan path aktual ke instalasi Java Anda:
export JAVA_HOME=/path/to/java
Simpan file dan mulai ulang terminal agar perubahan berlaku.
Setelah mengikuti langkah-langkah ini, Anda harus menginstal Java 8 atau versi yang lebih baru dan siap digunakan pada sistem Anda.
Langkah Integrasi
Proses ini melibatkan tiga langkah penting berikut:
- Rincian Pusat Pesan
- Menambahkan detail dalam kode
- Kirim SMS OTP tes
a. Rincian Pusat Pesan
Setelah membuat akun di Pusat Pesan, Anda memerlukan detail berikut:
- ID Pelanggan - Anda bisa mendapatkan ID pelanggan dari konsol rumah Message Central
- Kredensi Login: Anda memerlukan email dan perlu membuat kata sandi.
b. Menambahkan Detail dalam Kode
Untuk menambahkan detail MessageCentral pada kode cari tahu definisi variabel dengan nama “CustomerID”, “email” dan “password”:
private static final String customerID = "C-45539F39A44****";
private static final String email = "abhishek****k68@gmail.com";
private static final String password = "*****";
private static final String baseURL = "https://cpaas.messagecentral.com";
private static String authToken;
private static String verificationId;
Sekarang, Anda perlu membuat file JAVA yaitu Main.java: Dan tambahkan kode yang disediakan ke dalam file ini.
package u2opia.message central.verification;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.InetSocketAddress;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpServer;
import org.json.JSONObject;
public class Main {
private static final int PORT = 3000;
private static final String customerID = "C-45539F39A449437";
private static final String email = "abhishekkaushik68@gmail.com";
private static final String password = "Wesee@123";
private static final String baseURL = "https://cpaas.messagecentral.com";
private static String authToken;
private static String verificationId;
private static String parseJSON(String jsonString, String key) {
try {
JSONObject jsonObject = new JSONObject(jsonString);
if (jsonObject.has(key)) {return jsonObject.getString(key);}
} catch (Exception e) {e.printStackTrace();}
return null;
}
private static String generateAuthToken() {
try {
String base64String = Base64.getEncoder().encodeToString(password.getBytes(StandardCharsets.UTF_8));
String url = baseURL + "/auth/v1/authentication/token?country=IN&customerId=" + customerID + "&email=" + email + "&key=" + base64String + "&scope=NEW";
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("GET");
con.setRequestProperty("Accept", "*/*");
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
authToken = parseJSON(response, "token");
return authToken;
} else {System.out.println("Error generating auth token. Response code: " + responseCode);}
} catch (IOException e) {e.printStackTrace();}
return null;
}
private static String sendOtp(String countryCode, String mobileNumber) {
try {
String url = baseURL + "/verification/v3/send?countryCode=" + countryCode + "&customerId=" + customerID + "&flowType=SMS&mobileNumber=" + mobileNumber;
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Accept", "*/*");
con.setRequestProperty("authToken", authToken);
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
verificationId = parseJSON(parseJSON(response,"data"), "verificationId");
return verificationId;
} else {System.out.println("Error sending OTP. Response code: " + responseCode);}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
private static String validateOtp(String otpCode, String countryCode, String mobileNumber) {
try {
String url = baseURL + "/verification/v3/validateOtp?countryCode=" + countryCode + "&mobileNumber=" + mobileNumber + "&verificationId=" + verificationId + "&customerId=" + customerID + "&code=" + otpCode;
HttpURLConnection con = (HttpURLConnection) new URL(url).openConnection();
con.setRequestMethod("GET");
con.setRequestProperty("Accept", "*/*");
con.setRequestProperty("authToken", authToken);
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder responseBuilder = new StringBuilder();
while ((inputLine = in.readLine()) != null) {responseBuilder.append(inputLine);}
in.close();
String response = responseBuilder.toString();
String verificationStatus = parseJSON(response, "verificationStatus");
return verificationStatus;
} else {System.out.println("Error validating OTP. Response code: " + responseCode);}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
public static void main(String[] args) {
HttpServer server;
try {server = HttpServer.create(new InetSocketAddress(PORT), 0);}
catch (IOException e) {throw new RuntimeException(e);}
server.createContext("/sendotp/", new SendOtpHandler());
server.createContext("/validateOtp/", new ValidateOtpHandler());
server.start();
System.out.println("Server running at http://localhost:" + PORT);
}
static class SendOtpHandler implements HttpHandler {
@Override
public void handle(HttpExchange exchange) throws IOException {
if (exchange.getRequestMethod().equalsIgnoreCase("POST")) {
String[] params = exchange.getRequestURI().getPath().split("/");
String countryCode = params[2];
String mobileNumber = params[3];
generateAuthToken();
verificationId = sendOtp(countryCode, mobileNumber);
if (verificationId != null) {
String responseBody = "Otp sent! Successfully";
exchange.sendResponseHeaders(200, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
} else {
String responseBody = "Bad Request";
exchange.sendResponseHeaders(400, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
} else {
String responseBody = "Method not allowed";
exchange.sendResponseHeaders(405, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
}
}
static class ValidateOtpHandler implements HttpHandler {
@Override
public void handle(HttpExchange exchange) throws IOException {
if (exchange.getRequestMethod().equalsIgnoreCase("GET")) {
String[] params = exchange.getRequestURI().getPath().split("/");
String countryCode = params[2];
String mobileNumber = params[3];
String otpCode = params[4];
generateAuthToken();
String verificationStatus = validateOtp(otpCode, countryCode, mobileNumber);
if (verificationStatus != null && verificationStatus.equals("VERIFICATION_COMPLETED")) {
String responseBody = "Otp verification Done!";
exchange.sendResponseHeaders(200, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
} else {
String responseBody = "Bad Request";
exchange.sendResponseHeaders(400, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
} else {
String responseBody = "Method not allowed";
exchange.sendResponseHeaders(405, responseBody.length());
OutputStream os = exchange.getResponseBody();
os.write(responseBody.getBytes());
os.close();
}
}
}
}
c. Kirim SMS Tes OTP
Jika Anda perlu menguji layanan tanpa kode, Anda dapat pergi ke verifikasi SMS gratis halaman di situs web Pusat Pesan.
Untuk memastikan integrasi berhasil, kirim SMS OTP uji sebagai berikut:
1. Jalankan kode JAVA menggunakan perintah
Kompilasi file Java Anda menggunakan perintah javac.
javac Main.java
Jalankan program Java Anda menggunakan perintah java
java Main
2. Buka Postman dan atur Metode Permintaan sebagai POST dan
URL sebagai http://localhost:3000/sendotp/<countryCode>/<phone_number>
Inilah port 3000, itu default dan didefinisikan dalam kode, dapat diubah.
Contoh, Untuk URL Nomor Telepon India: http://localhost:3000/sendotp/91/123****123
- Gambar 1: Mengirim Otp
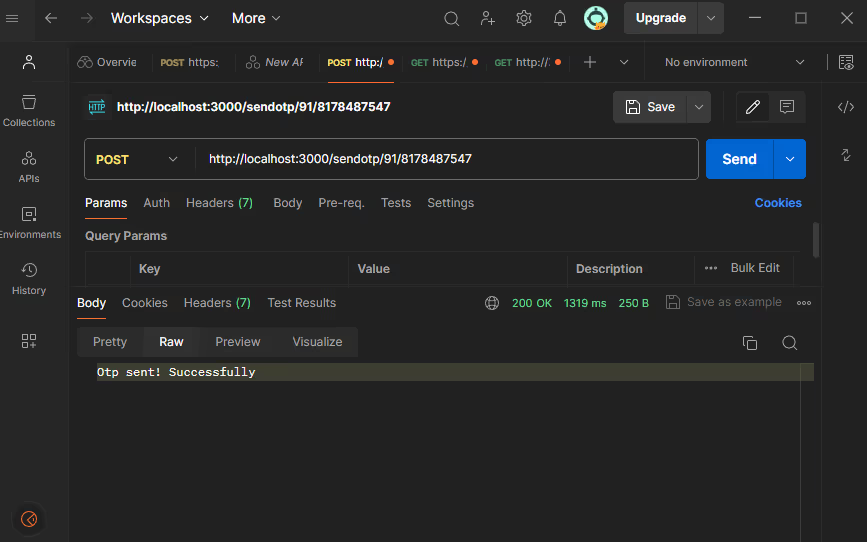
3. Sekarang Anda memiliki otp di kotak masuk sms Anda. Coba validasi Otp API Anda sendiri untuk memvalidasi OTP.
Buka Postman dan atur Metode Permintaan sebagai GET dan
URL sebagai http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Inilah port 3000, itu default dan didefinisikan dalam kode, dapat diubah.
Contoh, Untuk Nomor Telepon IndiaURL: http://localhost:3000/validateOtp/91/123****123/ ****
- Gambar 2: Memverifikasi Otp
