La configuración de la verificación por SMS
- Cree una cuenta de MessageCentral: Inscríbase en una central de mensajes cuenta para empezar. Recibirás un identificador de cliente, que utilizarás en tu solicitud.
- Instalación de C#
Descargar .NET SDK:
Ve a la página de descargas de.NET en el sitio web oficial de Microsoft.
Descargue la versión más reciente del SDK de.NET para su sistema operativo (Windows, macOS o Linux).
Ejecute el instalador:
Una vez finalizada la descarga, ejecuta el archivo de instalación.
Siga las instrucciones que aparecen en pantalla para instalar el SDK de.NET en su sistema.
Verifique la instalación:
Abra la línea de comandos (en Windows) o la terminal (en macOS/Linux).
Ejecute el siguiente comando para verificar la instalación:
dotnet --version
Esto debería imprimir la versión del SDK de.NET instalada. Si está instalado correctamente, deberías ver algo como:
5.0.403
(Opcional) Defina la variable de entorno PATH (Windows):
Haga clic con el botón derecho en «Este PC» o «Mi PC» y seleccione «Propiedades».
Haga clic en «Configuración avanzada del sistema» y luego en «Variables de entorno».
En la sección «Variables del sistema», seleccione la variable «Ruta» y haga clic en «Editar».
Agregue la ruta al directorio de instalación del SDK de.NET (p. ej., C:\Program Files\ dotnet) si aún no está presente.
Haga clic en «Aceptar» para guardar los cambios.
(Opcional) Establezca la variable de entorno PATH (macOS/Linux):
Abre una terminal y abre o crea el archivo.bash_profile en tu directorio principal.
Agregue la siguiente línea y sustituya /path/to/dotnet por la ruta real a la instalación del SDK de.NET:
export PATH=$PATH:/path/to/dotnet
MacOS/Linux:
Abre un editor de texto y crea o edita el archivo.bash_profile en tu directorio principal. Añada la siguiente línea y sustituya /path/to/java por la ruta real a la instalación de Java:
export JAVA_HOME=/path/to/java
Guarda el archivo y reinicia el terminal para que los cambios surtan efecto.
Tras seguir estos pasos, debería tener el SDK de.NET instalado y listo para usarse en el sistema.
Pasos de integración
Este proceso implica los siguientes tres pasos importantes:
- Detalles de Message Central
- Agregar detalles de Message Central en el código
- Enviar una opción de prueba para su verificación
un. Detalles de Message Central
Tras crear una cuenta en Message Central, necesitará los siguientes detalles:
- ID de cliente: puedes obtener el ID de cliente en Message Central Home Console
- Credenciales de inicio de sesión: necesitarás un correo electrónico y tendrás que crear una contraseña.
b. Añadir detalles de MessageCentral en el código
Para agregar detalles de MessageCentral en el código, busque la definición de la variable con el nombre «customerID», «correo electrónico» y «contraseña»:
private static string customerID = "C-45539F39A4*****";
private static string email = "abhishek*****k68@gmail.com";
private static string password = "*****";
private static string baseURL = "https://cpaas.messagecentral.com";
private static string authToken;
private static string verificationId;
private static string verificationStatus;
Ahora, debe crear un archivo de C# llamado Program.cs y agregar el código de C# proporcionado a este archivo.
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json.Linq;
namespace MessageCentralVerification
{
class Program
{
private static string customerID = "C-45539F39A4****";
private static string email = "abhishek***k68@gmail.com";
private static string password = "YOUR_PASSWORD";
private static string baseURL = "https://cpaas.messagecentral.com";
private static string authToken;
private static string verificationId;
private static string verificationStatus;
private static string ParseJSON(string jsonString, string key)
{
try
{
JObject jsonObject = JObject.Parse(jsonString);
return jsonObject.Value<string>(key);
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string GenerateAuthToken()
{
try
{
string base64String = Convert.ToBase64String(Encoding.UTF8.GetBytes(password));
string url = $"{baseURL}/auth/v1/authentication/token?country=IN&customerId={customerID}&email={email}&key={base64String}&scope=NEW";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "GET";
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
authToken = ParseJSON(responseString, "token");
return authToken;
}
}
else
{
Console.WriteLine($"Error generating auth token. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string SendOtp(string countryCode, string mobileNumber)
{
try
{
string url = $"{baseURL}/verification/v3/send?countryCode={countryCode}&customerId={customerID}&flowType=SMS&mobileNumber={mobileNumber}";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "POST";
request.Headers["authToken"] = authToken;
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
verificationId = ParseJSON(ParseJSON(responseString, "data"), "verificationId");
return verificationId;
}
}
else
{
Console.WriteLine($"Error sending OTP. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string ValidateOtp(string otpCode, string countryCode, string mobileNumber)
{
try
{
string url = $"{baseURL}/verification/v3/validateOtp?countryCode={countryCode}&mobileNumber={mobileNumber}&verificationId={verificationId}&customerId={customerID}&code={otpCode}";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "GET";
request.Headers["authToken"] = authToken;
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
verificationStatus = ParseJSON(responseString, "verificationStatus");
return verificationStatus;
}
}
else
{
Console.WriteLine($"Error validating OTP. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
static async Task Main(string[] args)
{
HttpListener listener = new HttpListener();
listener.Prefixes.Add("http://localhost:3000/");
listener.Start();
Console.WriteLine("Server running at http://localhost:3000/");
while (true)
{
HttpListenerContext context = await listener.GetContextAsync();
HttpListenerRequest request = context.Request;
HttpListenerResponse response = context.Response;
if (request.HttpMethod == "POST" && request.Url.AbsolutePath.StartsWith("/sendotp/"))
{
string[] pathSegments = request.Url.AbsolutePath.Split('/');
string countryCode = pathSegments[pathSegments.Length - 2];
string mobileNumber = pathSegments[pathSegments.Length - 1];
GenerateAuthToken();
verificationId = SendOtp(countryCode, mobileNumber);
string responseBody = verificationId != null ? "Otp sent! Successfully" : "Bad Request";
byte[] buffer = Encoding.UTF8.GetBytes(responseBody);
response.ContentLength64 = buffer.Length;
Stream output = response.OutputStream;
output.Write(buffer, 0, buffer.Length);
output.Close();
}
else if (request.HttpMethod == "GET" && request.Url.AbsolutePath.StartsWith("/validateOtp/"))
{
string[] pathSegments = request.Url.AbsolutePath.Split('/');
string countryCode = pathSegments[pathSegments.Length - 3];
string mobileNumber = pathSegments[pathSegments.Length - 2];
string otpCode = pathSegments[pathSegments.Length - 1];
GenerateAuthToken();
verificationStatus = ValidateOtp(otpCode, countryCode, mobileNumber);
string responseBody = verificationStatus == "VERIFICATION_COMPLETED" ? "Otp verification Done!" : "Bad Request";
byte[] buffer = Encoding.UTF8.GetBytes(responseBody);
response.ContentLength64 = buffer.Length;
Stream output = response.OutputStream;
output.Write(buffer, 0, buffer.Length);
output.Close();
}
else
{
response.StatusCode = 404;
response.Close();
}
}
}
}
}
c. Enviar un SMS de prueba de Otp para su verificación
Si necesita probar el servicio sin código, puede ir a verificación por SMS gratuita página en el sitio web de Message Central.
Para garantizar que la integración se realice correctamente, envíe un SMS OTP de prueba de la siguiente manera:
- Para ejecutar el código de C#, debe compilarlo y ejecutarlo con el SDK de.NET Core. Puedes hacerlo de la siguiente manera:
Compila tu archivo de C# con el comando csc (compilador de C#).
csc Program.cs
Ejecute el programa compilado de C# con el comando dotnet.
dotnet Program.dll
Asegúrese de tener el SDK de.NET Core instalado en el sistema. Si no lo tienes instalado, puedes descargarlo del sitio web oficial de .NET Core:
https://dotnet.microsoft.com/download
- Abra Postman y configure el método de solicitud como POST y
URL como http://localhost:3000/sendotp/<countryCode>/<phone_number>
Aquí está el puerto 3000, es predeterminado y está definido en código, se puede cambiar.
Ejemplo, para la URL de un número de teléfono indio: http://localhost:3000/sendotp/91/123****123
- Imagen 1: Sending Otp
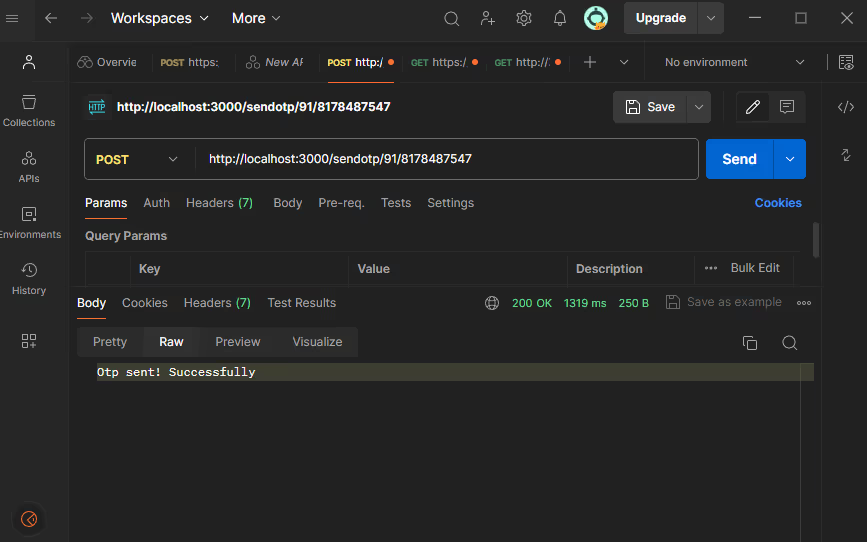
- Ahora tienes una OTP en tu bandeja de entrada de sms. Pruebe su propia API de validación de OTP para validar OTP.
Abra Postman y configure el método de solicitud como GET y
URL como http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Aquí está el puerto 3000, es predeterminado y está definido en código, se puede cambiar.
Por ejemplo, para la URL del número de teléfono indio: http://localhost:3000/validateOtp/91/123****123/ ****
- Imagen 2: Verificación de Otp
