Pengaturan untuk Verifikasi SMS
- Buat Akun MessageCentral: Mendaftar untuk Pusat Pesan akun untuk memulai. Anda akan menerima ID pelanggan, yang akan Anda gunakan dalam aplikasi Anda.
- Menginstal C #
Unduh SDK .NET:
Buka halaman unduhan.NET di situs web resmi Microsoft.
Unduh versi terbaru SDK.NET untuk sistem operasi Anda (Windows, macOS, atau Linux).
Jalankan Installer:
Setelah unduhan selesai, jalankan file penginstal.
Ikuti petunjuk di layar untuk menginstal.NET SDK pada sistem Anda.
Verifikasi Instalasi:
Buka command prompt (pada Windows) atau terminal (di MacOS/Linux).
Jalankan perintah berikut untuk memverifikasi instalasi:
dotnet --version
Ini harus mencetak versi SDK .NET yang diinstal. Jika diinstal dengan benar, Anda akan melihat sesuatu seperti:
5.0.403
(Opsional) Atur Variabel Lingkungan PATH (Windows):
Klik kanan pada “PC ini” atau “My Computer” dan pilih “Properties”.
Klik “Pengaturan sistem lanjutan” dan kemudian “Variabel Lingkungan”.
Di bagian “Variabel sistem”, pilih variabel “Path” dan klik “Edit”.
Tambahkan path ke direktori instalasi.NET SDK (misalnya, C:\Program Files\ dotnet) jika belum ada.
Klik “OK” untuk menyimpan perubahan.
(Opsional) Atur Variabel Lingkungan PATH (macOS/Linux):
Buka terminal dan buka atau buat file.bash_profile di direktori home Anda.
Tambahkan baris berikut, ganti /path/to/dotnet dengan path aktual ke instalasi .NET SDK Anda:
export PATH=$PATH:/path/to/dotnet
MacOS/Linux:
Buka editor teks dan buat atau edit file.bash_profile di direktori home Anda. Tambahkan baris berikut, ganti /path/to/java dengan path aktual ke instalasi Java Anda:
export JAVA_HOME=/path/to/java
Simpan file dan mulai ulang terminal agar perubahan berlaku.
Setelah mengikuti langkah-langkah ini, Anda harus menginstal.NET SDK dan siap digunakan pada sistem Anda.
Langkah Integrasi
Proses ini melibatkan tiga langkah penting berikut:
- Rincian Pusat Pesan
- Menambahkan Detail Pusat Pesan dalam Kode
- Kirim Test Otp untuk verifikasi
a. Rincian Pusat Pesan
Setelah membuat akun di Pusat Pesan, Anda memerlukan detail berikut:
- ID Pelanggan - Anda bisa mendapatkan ID pelanggan dari Message Central Home Console
- Kredensi Login: Anda memerlukan email dan perlu membuat kata sandi.
b. Menambahkan Detail MessageCentral dalam Kode
Untuk menambahkan detail MessageCentral pada kode cari tahu definisi variabel dengan nama “CustomerID”, “email” dan “password”:
private static string customerID = "C-45539F39A4*****";
private static string email = "abhishek*****k68@gmail.com";
private static string password = "*****";
private static string baseURL = "https://cpaas.messagecentral.com";
private static string authToken;
private static string verificationId;
private static string verificationStatus;
Sekarang, Anda perlu membuat file C # bernama Program.cs dan menambahkan kode C# yang disediakan ke dalam file ini.
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json.Linq;
namespace MessageCentralVerification
{
class Program
{
private static string customerID = "C-45539F39A4****";
private static string email = "abhishek***k68@gmail.com";
private static string password = "YOUR_PASSWORD";
private static string baseURL = "https://cpaas.messagecentral.com";
private static string authToken;
private static string verificationId;
private static string verificationStatus;
private static string ParseJSON(string jsonString, string key)
{
try
{
JObject jsonObject = JObject.Parse(jsonString);
return jsonObject.Value<string>(key);
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string GenerateAuthToken()
{
try
{
string base64String = Convert.ToBase64String(Encoding.UTF8.GetBytes(password));
string url = $"{baseURL}/auth/v1/authentication/token?country=IN&customerId={customerID}&email={email}&key={base64String}&scope=NEW";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "GET";
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
authToken = ParseJSON(responseString, "token");
return authToken;
}
}
else
{
Console.WriteLine($"Error generating auth token. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string SendOtp(string countryCode, string mobileNumber)
{
try
{
string url = $"{baseURL}/verification/v3/send?countryCode={countryCode}&customerId={customerID}&flowType=SMS&mobileNumber={mobileNumber}";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "POST";
request.Headers["authToken"] = authToken;
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
verificationId = ParseJSON(ParseJSON(responseString, "data"), "verificationId");
return verificationId;
}
}
else
{
Console.WriteLine($"Error sending OTP. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string ValidateOtp(string otpCode, string countryCode, string mobileNumber)
{
try
{
string url = $"{baseURL}/verification/v3/validateOtp?countryCode={countryCode}&mobileNumber={mobileNumber}&verificationId={verificationId}&customerId={customerID}&code={otpCode}";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "GET";
request.Headers["authToken"] = authToken;
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
verificationStatus = ParseJSON(responseString, "verificationStatus");
return verificationStatus;
}
}
else
{
Console.WriteLine($"Error validating OTP. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
static async Task Main(string[] args)
{
HttpListener listener = new HttpListener();
listener.Prefixes.Add("http://localhost:3000/");
listener.Start();
Console.WriteLine("Server running at http://localhost:3000/");
while (true)
{
HttpListenerContext context = await listener.GetContextAsync();
HttpListenerRequest request = context.Request;
HttpListenerResponse response = context.Response;
if (request.HttpMethod == "POST" && request.Url.AbsolutePath.StartsWith("/sendotp/"))
{
string[] pathSegments = request.Url.AbsolutePath.Split('/');
string countryCode = pathSegments[pathSegments.Length - 2];
string mobileNumber = pathSegments[pathSegments.Length - 1];
GenerateAuthToken();
verificationId = SendOtp(countryCode, mobileNumber);
string responseBody = verificationId != null ? "Otp sent! Successfully" : "Bad Request";
byte[] buffer = Encoding.UTF8.GetBytes(responseBody);
response.ContentLength64 = buffer.Length;
Stream output = response.OutputStream;
output.Write(buffer, 0, buffer.Length);
output.Close();
}
else if (request.HttpMethod == "GET" && request.Url.AbsolutePath.StartsWith("/validateOtp/"))
{
string[] pathSegments = request.Url.AbsolutePath.Split('/');
string countryCode = pathSegments[pathSegments.Length - 3];
string mobileNumber = pathSegments[pathSegments.Length - 2];
string otpCode = pathSegments[pathSegments.Length - 1];
GenerateAuthToken();
verificationStatus = ValidateOtp(otpCode, countryCode, mobileNumber);
string responseBody = verificationStatus == "VERIFICATION_COMPLETED" ? "Otp verification Done!" : "Bad Request";
byte[] buffer = Encoding.UTF8.GetBytes(responseBody);
response.ContentLength64 = buffer.Length;
Stream output = response.OutputStream;
output.Write(buffer, 0, buffer.Length);
output.Close();
}
else
{
response.StatusCode = 404;
response.Close();
}
}
}
}
}
c. Kirim SMS Test Otp untuk Verifikasi
Jika Anda perlu menguji layanan tanpa kode, Anda dapat pergi ke verifikasi SMS gratis halaman di situs web Pusat Pesan.
Untuk memastikan integrasi berhasil, kirim SMS OTP uji sebagai berikut:
- Untuk menjalankan kode C#, Anda perlu mengkompilasi dan menjalankannya menggunakan .NET Core SDK. Berikut cara Anda melakukannya:
Kompilasi file C# Anda menggunakan perintah csc (kompiler C#).
csc Program.cs
Jalankan program C# yang dikompilasi menggunakan perintah dotnet.
dotnet Program.dll
Pastikan Anda telah menginstal.NET Core SDK di sistem Anda. Jika Anda belum menginstalnya, Anda dapat mengunduhnya dari situs resmi.NET Core:
https://dotnet.microsoft.com/download
- Buka Postman dan atur Metode Permintaan sebagai POST dan
URL sebagai http://localhost:3000/sendotp/<countryCode>/<phone_number>
Inilah port 3000, itu default dan didefinisikan dalam kode, dapat diubah.
Contoh, Untuk URL Nomor Telepon India: http://localhost:3000/sendotp/91/123****123
- Gambar 1: Mengirim Otp
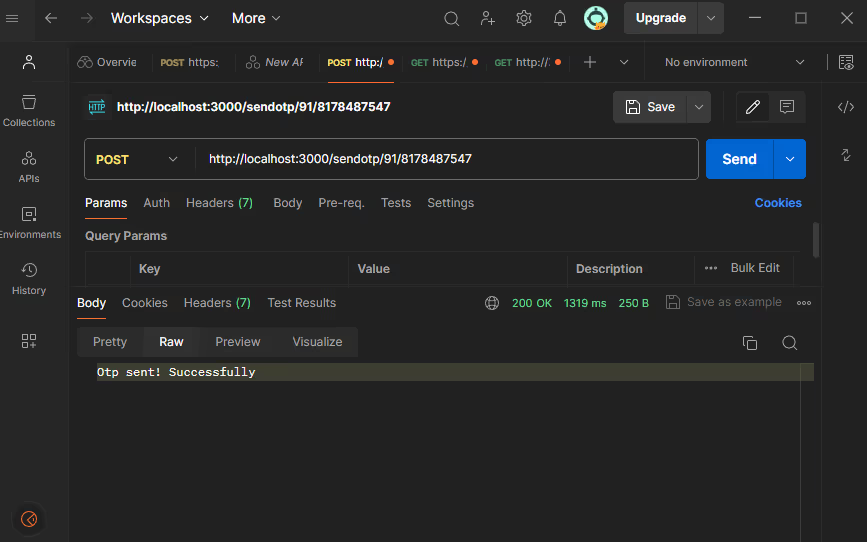
- Sekarang Anda memiliki otp di kotak masuk sms Anda. Coba validasi Otp API Anda sendiri untuk memvalidasi OTP.
Buka Postman dan atur Metode Permintaan sebagai GET dan
URL sebagai http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Inilah port 3000, itu default dan didefinisikan dalam kode, dapat diubah.
Contoh, Untuk Nomor Telepon IndiaURL: http://localhost:3000/validateOtp/91/123****123/ ****
- Gambar 2: Memverifikasi Otp
