The Setup for SMS Verification
- Create a MessageCentral Account: Sign up for a Message Central account to get started. You will receive a customer ID, which you will use in your application.
- Installing C#
Download .NET SDK:
Go to the .NET download page on the official Microsoft website.
Download the latest version of the .NET SDK for your operating system (Windows, macOS, or Linux).
Run the Installer:
Once the download is complete, run the installer file.
Follow the on-screen instructions to install the .NET SDK on your system.
Verify Installation:
Open the command prompt (on Windows) or terminal (on macOS/Linux).
Run the following command to verify the installation:
dotnet --version
This should print the installed .NET SDK version. If installed correctly, you should see something like:
5.0.403
(Optional) Set PATH Environment Variable (Windows):
Right-click on "This PC" or "My Computer" and select "Properties".
Click on "Advanced system settings" and then "Environment Variables".
In the "System variables" section, select the "Path" variable and click "Edit".
Add the path to the .NET SDK installation directory (e.g., C:\Program Files\dotnet) if it's not already present.
Click "OK" to save the changes.
(Optional) Set PATH Environment Variable (macOS/Linux):
Open a terminal and open or create the .bash_profile file in your home directory.
Add the following line, replacing /path/to/dotnet with the actual path to your .NET SDK installation:
export PATH=$PATH:/path/to/dotnet
macOS/Linux:
Open a text editor and create or edit the .bash_profile file in your home directory. Add the following line, replacing /path/to/java with the actual path to your Java installation:
export JAVA_HOME=/path/to/java
Save the file and restart the terminal for the changes to take effect.
After following these steps, you should have the .NET SDK installed and ready to use on your system.
Integration Steps
This process involves the following three significant steps:
- Message Central Details
- Adding Message Central Details in Code
- Send a Test Otp for verification
a. Message Central Details
After creating an account on Message Central, you need the following details:
- Customer Id - You can get the customer ID from Message Central Home Console
- Login Credentials: You’d require an email and would need to create a password.
b. Adding MessageCentral Details in Code
To add MessageCentral details on the code find out the variable definition with name “customerId”, “email” and “password”:
private static string customerID = "C-45539F39A4*****";
private static string email = "abhishek*****k68@gmail.com";
private static string password = "*****";
private static string baseURL = "https://cpaas.messagecentral.com";
private static string authToken;
private static string verificationId;
private static string verificationStatus;
Now, you need to create a C# file named Program.cs and add the provided C# code into this file.
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json.Linq;
namespace MessageCentralVerification
{
class Program
{
private static string customerID = "C-45539F39A4****";
private static string email = "abhishek***k68@gmail.com";
private static string password = "YOUR_PASSWORD";
private static string baseURL = "https://cpaas.messagecentral.com";
private static string authToken;
private static string verificationId;
private static string verificationStatus;
private static string ParseJSON(string jsonString, string key)
{
try
{
JObject jsonObject = JObject.Parse(jsonString);
return jsonObject.Value<string>(key);
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string GenerateAuthToken()
{
try
{
string base64String = Convert.ToBase64String(Encoding.UTF8.GetBytes(password));
string url = $"{baseURL}/auth/v1/authentication/token?country=IN&customerId={customerID}&email={email}&key={base64String}&scope=NEW";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "GET";
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
authToken = ParseJSON(responseString, "token");
return authToken;
}
}
else
{
Console.WriteLine($"Error generating auth token. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string SendOtp(string countryCode, string mobileNumber)
{
try
{
string url = $"{baseURL}/verification/v3/send?countryCode={countryCode}&customerId={customerID}&flowType=SMS&mobileNumber={mobileNumber}";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "POST";
request.Headers["authToken"] = authToken;
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
verificationId = ParseJSON(ParseJSON(responseString, "data"), "verificationId");
return verificationId;
}
}
else
{
Console.WriteLine($"Error sending OTP. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
private static string ValidateOtp(string otpCode, string countryCode, string mobileNumber)
{
try
{
string url = $"{baseURL}/verification/v3/validateOtp?countryCode={countryCode}&mobileNumber={mobileNumber}&verificationId={verificationId}&customerId={customerID}&code={otpCode}";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "GET";
request.Headers["authToken"] = authToken;
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string responseString = reader.ReadToEnd();
verificationStatus = ParseJSON(responseString, "verificationStatus");
return verificationStatus;
}
}
else
{
Console.WriteLine($"Error validating OTP. Response code: {response.StatusCode}");
return null;
}
}
}
catch (Exception e)
{
Console.WriteLine(e);
return null;
}
}
static async Task Main(string[] args)
{
HttpListener listener = new HttpListener();
listener.Prefixes.Add("http://localhost:3000/");
listener.Start();
Console.WriteLine("Server running at http://localhost:3000/");
while (true)
{
HttpListenerContext context = await listener.GetContextAsync();
HttpListenerRequest request = context.Request;
HttpListenerResponse response = context.Response;
if (request.HttpMethod == "POST" && request.Url.AbsolutePath.StartsWith("/sendotp/"))
{
string[] pathSegments = request.Url.AbsolutePath.Split('/');
string countryCode = pathSegments[pathSegments.Length - 2];
string mobileNumber = pathSegments[pathSegments.Length - 1];
GenerateAuthToken();
verificationId = SendOtp(countryCode, mobileNumber);
string responseBody = verificationId != null ? "Otp sent! Successfully" : "Bad Request";
byte[] buffer = Encoding.UTF8.GetBytes(responseBody);
response.ContentLength64 = buffer.Length;
Stream output = response.OutputStream;
output.Write(buffer, 0, buffer.Length);
output.Close();
}
else if (request.HttpMethod == "GET" && request.Url.AbsolutePath.StartsWith("/validateOtp/"))
{
string[] pathSegments = request.Url.AbsolutePath.Split('/');
string countryCode = pathSegments[pathSegments.Length - 3];
string mobileNumber = pathSegments[pathSegments.Length - 2];
string otpCode = pathSegments[pathSegments.Length - 1];
GenerateAuthToken();
verificationStatus = ValidateOtp(otpCode, countryCode, mobileNumber);
string responseBody = verificationStatus == "VERIFICATION_COMPLETED" ? "Otp verification Done!" : "Bad Request";
byte[] buffer = Encoding.UTF8.GetBytes(responseBody);
response.ContentLength64 = buffer.Length;
Stream output = response.OutputStream;
output.Write(buffer, 0, buffer.Length);
output.Close();
}
else
{
response.StatusCode = 404;
response.Close();
}
}
}
}
}
c. Send a Test Otp Sms for Verification
If you need to test the service without code, you can go to the free SMS verification page on the Message Central’s website.
To ensure that the integration is successful, send a test OTP SMS as follows:
- To run the C# code, you need to compile and execute it using the .NET Core SDK. Here's how you can do it:
Compile your C# file using the csc command (C# compiler).
csc Program.cs
Run your compiled C# program using the dotnet command.
dotnet Program.dll
Make sure you have the .NET Core SDK installed on your system. If you don't have it installed, you can download it from the official .NET Core website:
https://dotnet.microsoft.com/download
- Open the Postman and set Request Method as POST and
URL as http://localhost:3000/sendotp/<countryCode>/<phone_number>
Here’s the port 3000, it is default and defined in code, can be changed.
Example, For Indian Phone Number URL : http://localhost:3000/sendotp/91/123****123
- Image 1 : Sending Otp
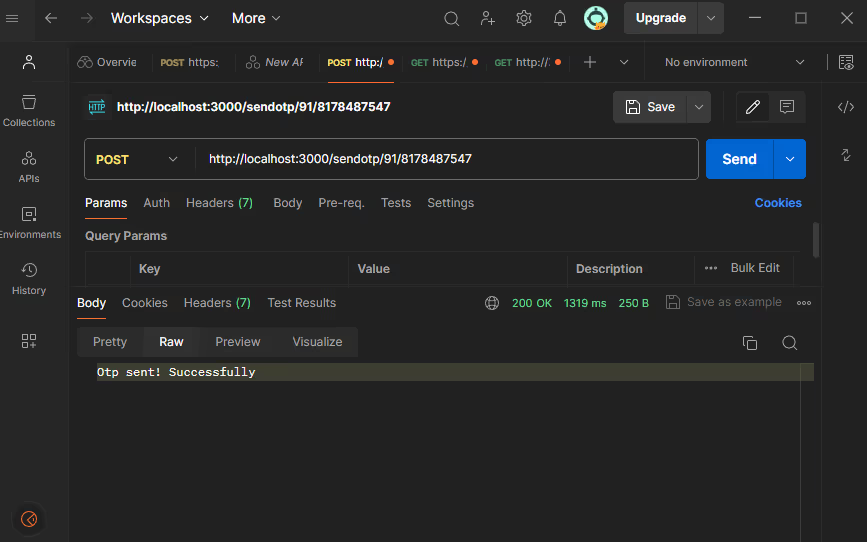
- Now You have an otp on your sms inbox. Try your own validation Otp API to validate OTP.
Open the Postman and set Request Method as GET and
URL as http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Here’s the port 3000, it is default and defined in code, can be changed.
Example, For Indian Phone NumberURL : http://localhost:3000/validateOtp/91/123****123/****
- Image 2 : Verifying Otp
