Pengaturan untuk Verifikasi SMS dengan Pusat Pesan
Pusat Pesan adalah solusi CPaaS dengan berbagai produk termasuk API SMS OTP, SMS API dan platform pemasaran WhatsApp. You Can buat akun gratis dan akan menerima ID pelanggan, yang akan Anda gunakan dalam aplikasi Anda.
Install Python
- Unduh Python:
Kunjungi situs web resmi Python (https://www.python.org/downloads/) dan unduh Python versi terbaru untuk sistem operasi Anda.
- Jalankan Installer:
Setelah pengunduhan selesai, jalankan file penginstalan. Ikuti petunjuk di layar untuk menginstal Python di sistem Anda. Pastikan untuk memasukkan kotak yang bertuliskan “Tambahkan Python ke PATH” selama instalasi.
- Verifikasi Instalasi:
Buka command prompt (pada Windows) atau terminal (di macOS/Linux) dan jalankan perintah berikut untuk menginstal lebih awal:
python --version
Ini harus mencetak versi Python yang tidak diinstal. Jika Python diinstal dengan benar, Anda akan melihat sesuatu seperti:
Python 3.10.1
- Setel Variabel PATH (hanya Windows):
Jika Python tidak dikenal sebagai perintah, Anda mungkin perlu memasukkannya ke variabel lingkungan PATH secara manual. Untuk melakukan ini, ikuti langkah-langkah ini:
Klik kanan pada “My Computer” dan pilih “Properties”.
Klik “Pengaturan sistem lanjutan” > “Variabel Lingkungan”.
Di bawah “Variabel sistem”, temukan variabel “Path” dan klik “Edit”.
Klik “Baru” dan tambahkan jalur ke instalasi Python Anda (misalnya, C:\Python310) dan klik “OK”.
- Verifikasi Instalasi Python:
Tutup dan buka kembali command prompt (atau terminal) dan jalankan python --version lagi untuk memastikan bahwa Python sekarang terdengar sebagai perintah.
Setelah mengikuti langkah ini, Anda harus menginstal Python dan siap digunakan pada sistem Anda.
Perlengkapan untuk mengintegrasikan
Proses ini melibatkan hal-hal berikut:
- Mendapatkan rincian Pusat Pesan
- Menambahkan Detail Pusat Pesan dalam Kode
- Kirim Tes OTP untuk verifikasi
a. rincian Pusat Pesan.
Setelah membuat akun di Pusat Pesan, Anda memerlukan detail berikut:
- ID Pelanggan - Anda bisa mendapatkan ID pelanggan dari Message Central Home Console
- Kredit Login: Anda memerlukan email dan perlu membuat kata sandi.
Menambahkan Detail Pusat Pesan dalam Kode
Untuk menambahkan detail MessageCentral pada kode cari tahu definisi variabel dengan nama “CustomerID”, “email” dan “password”:
customer_id = "C-45539F39A44****"
email = "abhishek*****k68@gmail.com"
password = "*******"
base_url = "https://cpaas.messagecentral.com"
auth_token = None
verification_id = None
verification_status = None
Sekarang, Anda perlu membuat file Python yaitu Main.py: Kemudian tambahkan kode yang disediakan dalam file ini.
from http.server import BaseHTTPRequestHandler, HTTPServer
from urllib.parse import urlparse, parse_qs
import json
import requests
import base64
customer_id = "C-45539F39A44****"
email = "abhishek*****k68@gmail.com"
password = "*****"
base_url = "https://cpaas.messagecentral.com"
auth_token = None
verification_id = None
verification_status = None
def generate_auth_token():
global auth_token
print(auth_token)
try:
base64_string = base64.b64encode(password.encode('utf-8')).decode('utf-8')
url = f"{base_url}/auth/v1/authentication/token?country=IN&customerId={customer_id}&email={email}&key={base64_string}&scope=NEW"
response = requests.get(url)
if response.status_code == 200:
auth_token = json.loads(response.text)["token"]
return auth_token
else:
print(f"Error generating auth token. Response code: {response.status_code}")
except Exception as e:
print(f"Exception: {e}")
return None
def send_otp(country_code, mobile_number):
global auth_token, verification_id
print(auth_token)
print(verification_id)
try:
url = f"{base_url}/verification/v3/send?countryCode={country_code}&customerId={customer_id}&flowType=SMS&mobileNumber={mobile_number}"
headers = {
"Accept": "*/*",
"authToken": auth_token
}
response = requests.post(url, headers=headers)
print(response.text)
if response.status_code == 200:
response_json = json.loads(response.text)
verification_id = response_json.get("data", {}).get("verificationId")
return verification_id
else:
print(f"Error sending OTP. Response code: {response.status_code}")
except Exception as e:
print(f"Exception: {e}")
return None
def validate_otp(otp_code, country_code, mobile_number):
global auth_token, verification_id, verification_status
print(auth_token)
print(verification_id)
print(verification_status)
try:
url = f"{base_url}/verification/v3/validateOtp?countryCode={country_code}&mobileNumber={mobile_number}&verificationId={verification_id}&customerId={customer_id}&code={otp_code}"
print(url)
headers = {
"Accept": "*/*",
"authToken": auth_token
}
response = requests.get(url, headers=headers)
print(response.text)
print(verification_id)
print(otp_code)
if response.status_code == 200:
verification_status = json.loads(response.text)["data"]["verificationStatus"]
print(verification_status)
return verification_status
else:
print(f"Error validating OTP. Response code: {response.status_code}")
except Exception as e:
print(f"Exception: {e}")
return None
class MyHTTPHandler(BaseHTTPRequestHandler):
def do_POST(self):
parsed_path = urlparse(self.path)
path = parsed_path.path
query = parse_qs(parsed_path.query)
if path.startswith("/sendotp/"):
country_code = path.split("/")[-2]
mobile_number = path.split("/")[-1]
generate_auth_token()
print(country_code)
print(mobile_number)
send_otp(country_code, mobile_number)
if verification_id is not None :
self.send_response(200)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(f"Otp sent! Successfully".encode())
else :
self.send_response(400)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(f"Bad Request".encode())
else:
self.send_response(404)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write("Not Found".encode())
def do_GET(self):
global verification_status
print(verification_status)
parsed_path = urlparse(self.path)
path = parsed_path.path
query = parse_qs(parsed_path.query)
if path.startswith("/validateOtp/"):
country_code = path.split("/")[-3]
mobile_number = path.split("/")[-2]
otp = path.split("/")[-1]
print(otp)
print(mobile_number)
print(country_code)
generate_auth_token()
validate_otp(otp,country_code, mobile_number)
if verification_status is not None and verification_status=="VERIFICATION_COMPLETED":
self.send_response(200)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(f"Otp verification Done!".encode())
else :
self.send_response(400)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(f"Bad Request".encode())
else:
self.send_response(404)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write("Not Found".encode())
def run(server_class=HTTPServer, handler_class=MyHTTPHandler, port=3000):
server_address = ('', port)
httpd = server_class(server_address, handler_class)
print(f"Starting server on port {port}")
httpd.serve_forever()
if __name__ == "__main__":
run()
c. Kirim SMS Tes OTP untuk Verifikasi
Jika Anda perlu menguji layanan tanpa kode, Anda dapat pergi ke verifikasi SMS gratis halaman di situs web Pusat Pesan.
Untuk memastikan integrasi yang berhasil, kirim SMS OTP uji sebagai berikut:
- Jalankan kode Python menggunakan perintah
Kompilasi dan jalankan file python Anda menggunakan perintah python.
python Main.py
- Buka Postman dan atur Metode Permintaan sebagai POST dan
URL sebagai http://localhost:3000/sendotp/<countryCode>/<phone_number>
Ini port 3000, default dan didefinisikan dalam kode, dapat diubah.
Contoh, Untuk URL Nomor Telepon India: http://localhost:3000/sendotp/91/123****123
- Gambar 1: Mengirim Otp
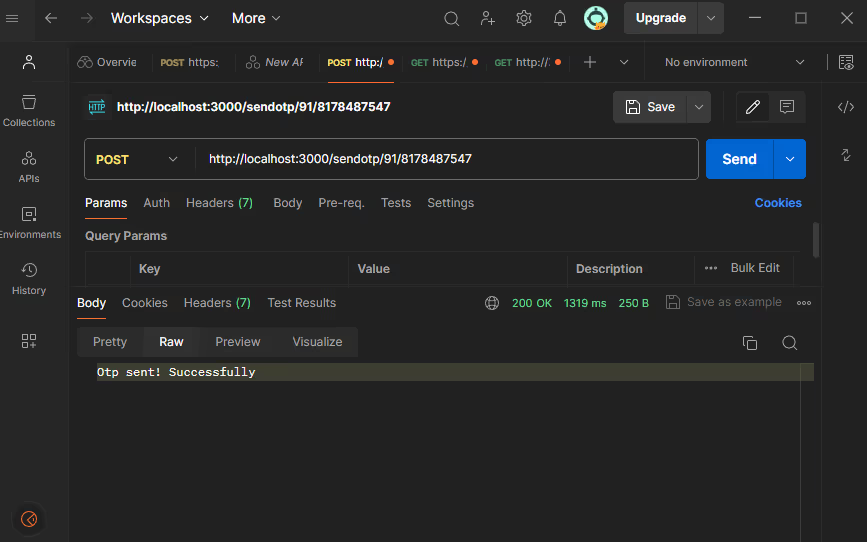
- Sekarang Anda memiliki otp di kotak masuk sms Anda. Coba validasi API Otp Anda sendiri untuk memvalidasi OTP.
Buka Postman dan atur Metode Permintaan sebagai GET dan
URL sebagai http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Ini port 3000, default dan didefinisikan dalam kode, dapat diubah.
Contoh, Untuk Nomor Telepon IndiaURL: http://localhost:3000/validateOtp/91/123****123/ ****- Gambar 2: Bawah Otp
