Setup for SMS Verification with Message Central
Message Central is a CPaaS solution with a suite of products including OTP SMS APIs, SMS APIs and WhatsApp marketing platform. You can create an account for free and will receive a customer ID, which you will use in your application.
Installing Python
- Download Python:
Visit the official Python website (https://www.python.org/downloads/) and download the latest version of Python for your operating system.
- Run the Installer:
Once the download is complete, run the installer file. Follow the on-screen instructions to install Python on your system. Make sure to check the box that says "Add Python to PATH" during installation.
- Verify Installation:
Open the command prompt (on Windows) or terminal (on macOS/Linux) and run the following command to verify the installation:
python --version
This should print the installed Python version. If Python is installed correctly, you should see something like:
Python 3.10.1
- Set PATH Variable (Windows only):
If Python is not recognized as a command, you may need to add it to the PATH environment variable manually. To do this, follow these steps:
Right-click on "My Computer" and select "Properties".
Click on "Advanced system settings" > "Environment Variables".
Under "System variables", find the "Path" variable and click "Edit".
Click "New" and add the path to your Python installation (e.g., C:\Python310) and click "OK".
- Verify Python Installation:
Close and reopen the command prompt (or terminal) and run python --version again to verify that Python is now recognized as a command.
After following these steps, you should have Python installed and ready to use on your system.
Steps to Integrate
This process involves the following the following steps:
- Getting Message Central details
- Adding Message Central Details in Code
- Send a Test OTP for verification
a. Message Central Details.
After creating an account on Message Central, you need the following details:
- Customer Id - You can get the customer ID from Message Central Home Console
- Login Credentials: You’d require an email and would need to create a password.
Adding Message Central Details in Code
To add MessageCentral details on the code find out the variable definition with name “customerId”, “email” and “password”:
customer_id = "C-45539F39A44****"
email = "abhishek*****k68@gmail.com"
password = "*******"
base_url = "https://cpaas.messagecentral.com"
auth_token = None
verification_id = None
verification_status = None
Now, you need to create a Python file i.e Main.py: And add provided code into this file.
from http.server import BaseHTTPRequestHandler, HTTPServer
from urllib.parse import urlparse, parse_qs
import json
import requests
import base64
customer_id = "C-45539F39A44****"
email = "abhishek*****k68@gmail.com"
password = "*****"
base_url = "https://cpaas.messagecentral.com"
auth_token = None
verification_id = None
verification_status = None
def generate_auth_token():
global auth_token
print(auth_token)
try:
base64_string = base64.b64encode(password.encode('utf-8')).decode('utf-8')
url = f"{base_url}/auth/v1/authentication/token?country=IN&customerId={customer_id}&email={email}&key={base64_string}&scope=NEW"
response = requests.get(url)
if response.status_code == 200:
auth_token = json.loads(response.text)["token"]
return auth_token
else:
print(f"Error generating auth token. Response code: {response.status_code}")
except Exception as e:
print(f"Exception: {e}")
return None
def send_otp(country_code, mobile_number):
global auth_token, verification_id
print(auth_token)
print(verification_id)
try:
url = f"{base_url}/verification/v3/send?countryCode={country_code}&customerId={customer_id}&flowType=SMS&mobileNumber={mobile_number}"
headers = {
"Accept": "*/*",
"authToken": auth_token
}
response = requests.post(url, headers=headers)
print(response.text)
if response.status_code == 200:
response_json = json.loads(response.text)
verification_id = response_json.get("data", {}).get("verificationId")
return verification_id
else:
print(f"Error sending OTP. Response code: {response.status_code}")
except Exception as e:
print(f"Exception: {e}")
return None
def validate_otp(otp_code, country_code, mobile_number):
global auth_token, verification_id, verification_status
print(auth_token)
print(verification_id)
print(verification_status)
try:
url = f"{base_url}/verification/v3/validateOtp?countryCode={country_code}&mobileNumber={mobile_number}&verificationId={verification_id}&customerId={customer_id}&code={otp_code}"
print(url)
headers = {
"Accept": "*/*",
"authToken": auth_token
}
response = requests.get(url, headers=headers)
print(response.text)
print(verification_id)
print(otp_code)
if response.status_code == 200:
verification_status = json.loads(response.text)["data"]["verificationStatus"]
print(verification_status)
return verification_status
else:
print(f"Error validating OTP. Response code: {response.status_code}")
except Exception as e:
print(f"Exception: {e}")
return None
class MyHTTPHandler(BaseHTTPRequestHandler):
def do_POST(self):
parsed_path = urlparse(self.path)
path = parsed_path.path
query = parse_qs(parsed_path.query)
if path.startswith("/sendotp/"):
country_code = path.split("/")[-2]
mobile_number = path.split("/")[-1]
generate_auth_token()
print(country_code)
print(mobile_number)
send_otp(country_code, mobile_number)
if verification_id is not None :
self.send_response(200)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(f"Otp sent! Successfully".encode())
else :
self.send_response(400)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(f"Bad Request".encode())
else:
self.send_response(404)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write("Not Found".encode())
def do_GET(self):
global verification_status
print(verification_status)
parsed_path = urlparse(self.path)
path = parsed_path.path
query = parse_qs(parsed_path.query)
if path.startswith("/validateOtp/"):
country_code = path.split("/")[-3]
mobile_number = path.split("/")[-2]
otp = path.split("/")[-1]
print(otp)
print(mobile_number)
print(country_code)
generate_auth_token()
validate_otp(otp,country_code, mobile_number)
if verification_status is not None and verification_status=="VERIFICATION_COMPLETED":
self.send_response(200)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(f"Otp verification Done!".encode())
else :
self.send_response(400)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write(f"Bad Request".encode())
else:
self.send_response(404)
self.send_header('Content-type', 'text/plain')
self.end_headers()
self.wfile.write("Not Found".encode())
def run(server_class=HTTPServer, handler_class=MyHTTPHandler, port=3000):
server_address = ('', port)
httpd = server_class(server_address, handler_class)
print(f"Starting server on port {port}")
httpd.serve_forever()
if __name__ == "__main__":
run()
c. Send a Test OTP SMS for Verification
If you need to test the service without code, you can go to the free SMS verification page on the Message Central’s website.
To ensure that the integration is successful, send a test OTP SMS as follows:
- Run the Python code using command
Compile and Run your python file using the python command.
python Main.py
- Open the Postman and set Request Method as POST and
URL as http://localhost:3000/sendotp/<countryCode>/<phone_number> Here’s the port 3000, it is default and defined in code, can be changed.
Example, For Indian Phone Number URL : http://localhost:3000/sendotp/91/123****123
- Image 1 : Sending Otp
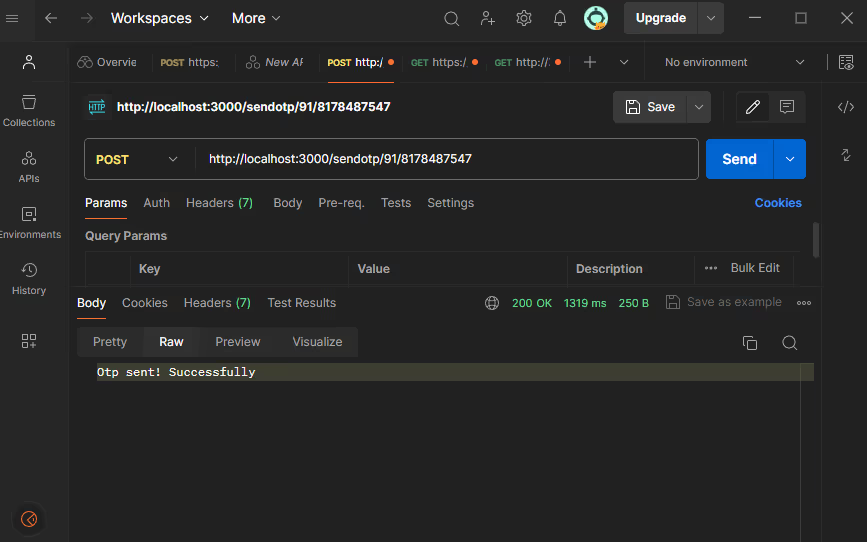
- Now You have an otp on your sms inbox. Try your own validation Otp API to validate OTP.
Open the Postman and set Request Method as GET and
URL as http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Here’s the port 3000, it is default and defined in code, can be changed.
Example, For Indian Phone NumberURL : http://localhost:3000/validateOtp/91/123****123/****- Image 2 : Verifying Otp
