Setup Your Account for SMS Verification
a. Create a MessageCentral Account: Sign up for a Message Central account to get started. You will receive a customer ID, which you will use in your application.
b. To install Ruby, you can follow these steps:
Download Ruby:
Visit the official Ruby download page: https://www.ruby-lang.org/en/downloads/
Download the latest version of Ruby for your operating system (Windows, macOS, or Linux).
Install Ruby:
Windows: Double-click the downloaded installer and follow the on-screen instructions.
macOS: Open the downloaded package and follow the installation instructions.
Linux: Use your package manager to install Ruby. For example, on Ubuntu, you can use
sudo apt-get update
sudo apt-get install ruby-full
Verify Installation:
Open a command prompt (on Windows) or terminal (on macOS/Linux).
Run the following command to verify the installation:
ruby --version
This should print the installed Ruby version. If installed correctly, you should see something like:
ruby 3.1.0p0 (2021-12-25 revision abcdef1234567890)
Once Ruby is installed, you can start using it to develop Ruby applications.
To set the PATH environment variable for Ruby, you can follow these steps:
Windows:
Right-click on "This PC" or "My Computer" and select "Properties".
Click on "Advanced system settings" and then "Environment Variables".
In the "System variables" section, select the "Path" variable and click "Edit".
Add the path to the Ruby executable directory (e.g., C:\Ruby27\bin) if it's not already present.
Click "OK" to save the changes.
macOS/Linux:
Open a terminal.
Open or create the .bash_profile file in your home directory.
nano ~/.bash_profile
Add the following line, replacing /path/to/ruby with the actual path to your Ruby executable directory:
export PATH=$PATH:/path/to/ruby
Save the file and exit the text editor.
Source the .bash_profile file to apply the changes:
After following these steps, the Ruby executable should be accessible from anywhere in your terminal session.
Integration Steps
This process involves the following three significant steps:
- Message Central Details
- Adding Message Central Details in Code
- Send a Test Otp for verification
a. Message Central Details
After creating an account on Message Central, you need the following details
- Customer Id - You can get the customer ID from Message Central Home Console
- Login Credentials: You’d require an email and would need to create a password
b. Adding MessageCentral Details in Code
To add MessageCentral details on the code find out the variable definition with name “customerId”, “email” and “password”:
customer_id = "C-45539F39A4*****"
email = "abhishek*****k68@gmail.com"
password = "*****"
base_url = "https://cpaas.messagecentral.com"
auth_token = nil
verification_id = nil
verification_status = nil
Now, you need to create a Ruby file named program.rb and add the provided Ruby code into this file.
require 'json'
require 'net/http'
customer_id = "C-45539F39A449437"
email = "abhishekkaushik68@gmail.com"
password = "Wesee@123"
base_url = "https://cpaas.messagecentral.com"
auth_token = nil
verification_id = nil
verification_status = nil
def parse_json(json_string, key)
begin
json = JSON.parse(json_string)
return json[key]
rescue JSON::ParserError => e
puts e
return nil
end
end
def generate_auth_token(customer_id, email, password, base_url)
begin
base64_string = Base64.strict_encode64(password)
url = "#{base_url}/auth/v1/authentication/token?country=IN&customerId=#{customer_id}&email=#{email}&key=#{base64_string}&scope=NEW"
uri = URI(url)
response = Net::HTTP.get_response(uri)
if response.code == '200'
auth_token = parse_json(response.body, 'token')
return auth_token
else
puts "Error generating auth token. Response code: #{response.code}"
return nil
end
rescue StandardError => e
puts e
return nil
end
end
def send_otp(country_code, mobile_number, base_url, auth_token)
begin
url = "#{base_url}/verification/v3/send?countryCode=#{country_code}&customerId=#{customer_id}&flowType=SMS&mobileNumber=#{mobile_number}"
uri = URI(url)
request = Net::HTTP::Post.new(uri)
request['Accept'] = '*/*'
request['authToken'] = auth_token
response = Net::HTTP.start(uri.hostname, uri.port, use_ssl: uri.scheme == 'https') do |http|
http.request(request)
end
if response.code == '200'
verification_id = parse_json(parse_json(response.body, 'data'), 'verificationId')
return verification_id
else
puts "Error sending OTP. Response code: #{response.code}"
return nil
end
rescue StandardError => e
puts e
return nil
end
end
def validate_otp(otp_code, country_code, mobile_number, verification_id, base_url, auth_token)
begin
url = "#{base_url}/verification/v3/validateOtp?countryCode=#{country_code}&mobileNumber=#{mobile_number}&verificationId=#{verification_id}&customerId=#{customer_id}&code=#{otp_code}"
uri = URI(url)
request = Net::HTTP::Get.new(uri)
request['Accept'] = '*/*'
request['authToken'] = auth_token
response = Net::HTTP.start(uri.hostname, uri.port, use_ssl: uri.scheme == 'https') do |http|
http.request(request)
end
if response.code == '200'
verification_status = parse_json(response.body, 'verificationStatus')
return verification_status
else
puts "Error validating OTP. Response code: #{response.code}"
return nil
end
rescue StandardError => e
puts e
return nil
end
end
def run_server(customer_id, email, password, base_url)
server = TCPServer.new('localhost', 3000)
puts "Server running at http://localhost:3000/"
loop do
Thread.start(server.accept) do |client|
request = client.gets
method, path = request.split(' ')
path_segments = path.split('/')
if method == 'POST' && path.start_with?('/sendotp/')
country_code = path_segments[-2]
mobile_number = path_segments[-1]
auth_token = generate_auth_token(customer_id, email, password, base_url)
verification_id = send_otp(country_code, mobile_number, base_url, auth_token)
response_body = verification_id ? 'Otp sent! Successfully' : 'Bad Request'
client.puts "HTTP/1.1 200 OK"
client.puts "Content-Type: text/plain"
client.puts
client.puts response_body
elsif method == 'GET' && path.start_with?('/validateOtp/')
country_code = path_segments[-3]
mobile_number = path_segments[-2]
otp_code = path_segments[-1]
auth_token = generate_auth_token(customer_id, email, password, base_url)
verification_status = validate_otp(otp_code, country_code, mobile_number, verification_id, base_url, auth_token)
response_body = verification_status == 'VERIFICATION_COMPLETED' ? 'Otp verification Done!' : 'Bad Request'
client.puts "HTTP/1.1 200 OK"
client.puts "Content-Type: text/plain"
client.puts
client.puts response_body
else
client.puts "HTTP/1.1 404 Not Found"
client.puts "Content-Type: text/plain"
client.puts
client.puts "Not Found"
end
client.close
end
end
end
run_server(customer_id, email, password, base_url)
c. Send a Test Otp Sms for Verification
If you need to test the service without code, you can go to the free SMS verification page on the Message Central’s website.
To ensure that the integration is successful, send a test OTP SMS as follows:
- To run the Ruby code, you need to execute it using the Ruby interpreter. Here's how you can do it:
Save your Ruby code in a file named program.rb.
Open a terminal or command prompt.
Navigate to the directory where program.rb is saved.
Run the Ruby file using the ruby command:ruby program.rb
This will execute your Ruby program and display any output it produces.
- Open the Postman and set Request Method as POST and
URL as http://localhost:3000/sendotp/<countryCode>/<phone_number>
Here’s the port 3000, it is default and defined in code, can be changed.
Example, For Indian Phone Number URL : http://localhost:3000/sendotp/91/123****123- Image 1: Sending Otp
- Image 1: Sending Otp
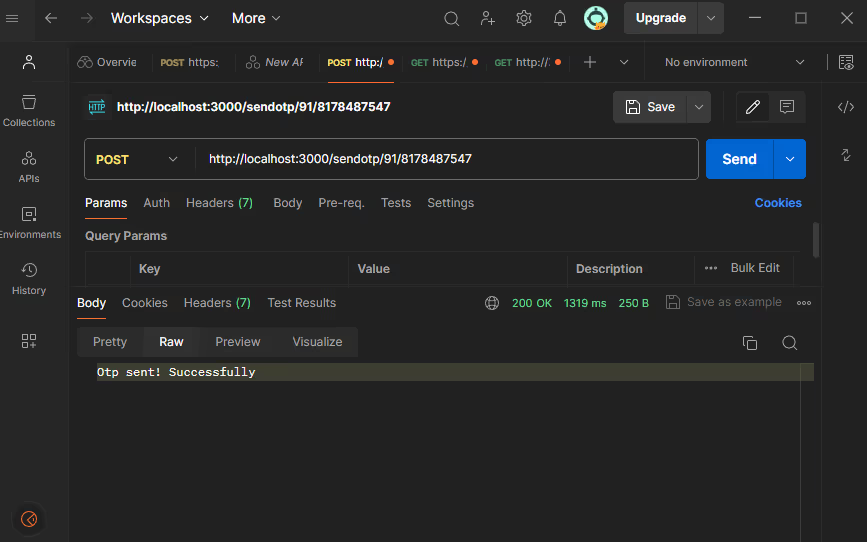
- Now You have an otp on your sms inbox. Try your own validation Otp API to validate OTP.
Open the Postman and set Request Method as GET and
URL as http://localhost:3000/validateOtp/<countryCode>/<phone_number>/<otp>
Here’s the port 3000, it is default and defined in code, can be changed.
Example, For Indian Phone NumberURL : http://localhost:3000/validateOtp/91/123****123/****
- Image 2 : Verifying Otp
- Image 2 : Verifying Otp
